Slow but Easy Text Drawing#
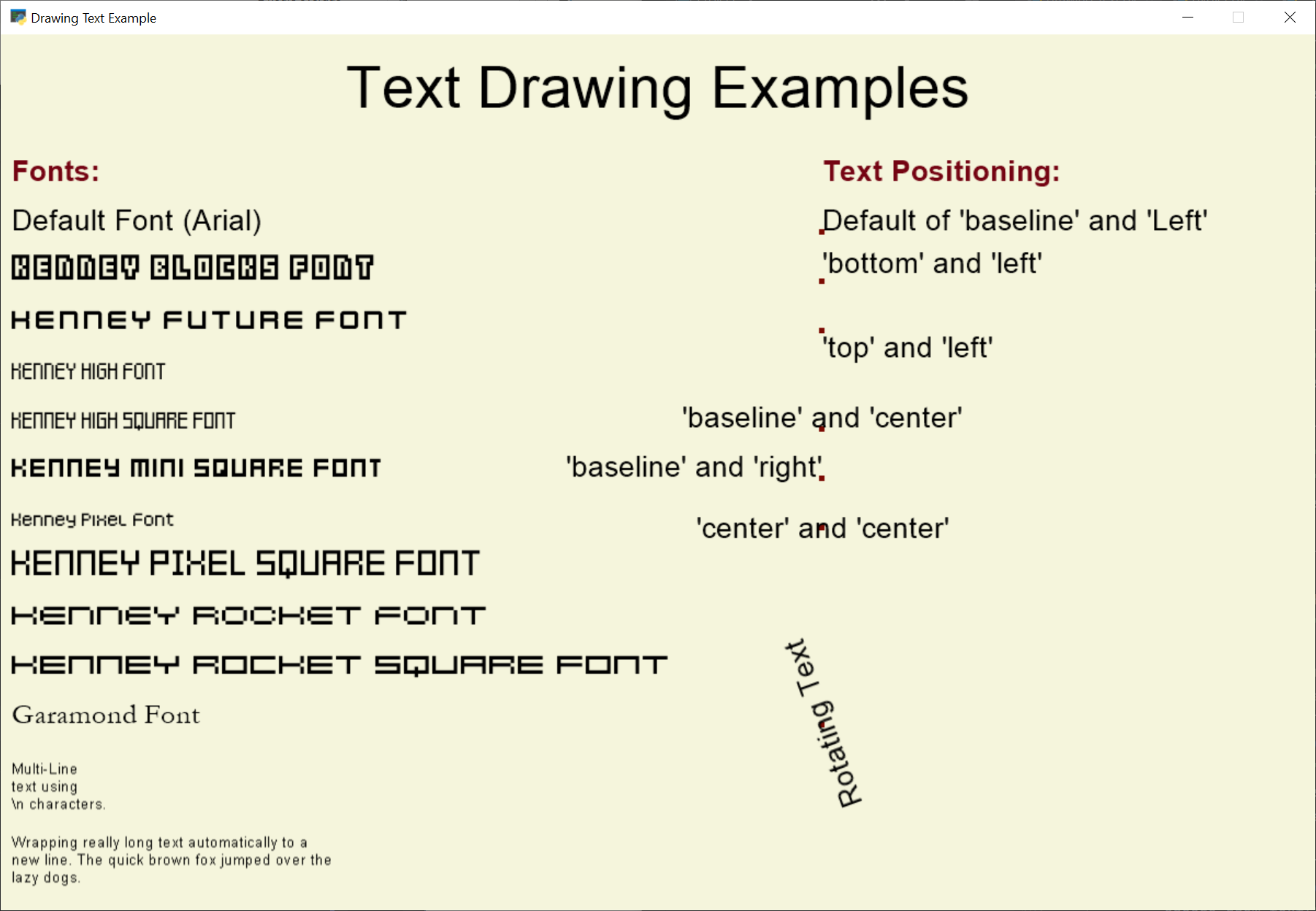
This example shows how to draw text. While it is simple to draw using the techniques shown here, it is also slow. Using “text objects” can result in significant performance improvements. See Fast Text Drawing.
drawing_text.py#
1"""
2Example showing how to draw text to the screen.
3
4If Python and Arcade are installed, this example can be run from the command line with:
5python -m arcade.examples.drawing_text
6"""
7import arcade
8
9SCREEN_WIDTH = 1200
10SCREEN_HEIGHT = 800
11SCREEN_TITLE = "Drawing Text Example"
12DEFAULT_LINE_HEIGHT = 45
13DEFAULT_FONT_SIZE = 20
14
15
16class MyGame(arcade.Window):
17 """
18 Main application class.
19 """
20
21 def __init__(self, width, height, title):
22 super().__init__(width, height, title)
23
24 self.background_color = arcade.color.BEIGE
25 self.text_angle = 0
26 self.time_elapsed = 0.0
27
28 def on_update(self, delta_time):
29 self.text_angle += 1
30 self.time_elapsed += delta_time
31
32 def on_draw(self):
33 """
34 Render the screen.
35 """
36
37 # This command should happen before we start drawing. It will clear
38 # the screen to the background color, and erase what we drew last frame.
39 self.clear()
40
41 # Add the screen title
42 start_x = 0
43 start_y = SCREEN_HEIGHT - DEFAULT_LINE_HEIGHT * 1.5
44 arcade.draw_text("Text Drawing Examples",
45 start_x,
46 start_y,
47 arcade.color.BLACK,
48 DEFAULT_FONT_SIZE * 2,
49 width=SCREEN_WIDTH,
50 align="center")
51
52 # start_x and start_y make the start point for the text. We draw a dot to make it
53 # easy to see the text in relation to its start x and y.
54 start_x = 10
55 start_y = SCREEN_HEIGHT - DEFAULT_LINE_HEIGHT * 3
56 arcade.draw_text("Fonts:",
57 start_x,
58 start_y,
59 arcade.color.FRENCH_WINE,
60 DEFAULT_FONT_SIZE, bold=True)
61
62 # Move the y value down to create another line of text
63 start_y -= DEFAULT_LINE_HEIGHT
64 arcade.draw_text("Default Font (Arial)",
65 start_x,
66 start_y,
67 arcade.color.BLACK,
68 DEFAULT_FONT_SIZE)
69
70 # Show some built-in fonts
71 start_y -= DEFAULT_LINE_HEIGHT
72 arcade.draw_text("Kenney Blocks Font",
73 start_x,
74 start_y,
75 arcade.color.BLACK,
76 DEFAULT_FONT_SIZE,
77 font_name="Kenney Blocks")
78
79 start_y -= DEFAULT_LINE_HEIGHT
80 arcade.draw_text("Kenney Future Font",
81 start_x,
82 start_y,
83 arcade.color.BLACK,
84 DEFAULT_FONT_SIZE,
85 font_name="Kenney Future")
86
87 start_y -= DEFAULT_LINE_HEIGHT
88 arcade.draw_text("Kenney High Font",
89 start_x,
90 start_y,
91 arcade.color.BLACK,
92 DEFAULT_FONT_SIZE,
93 font_name="Kenney High")
94
95 start_y -= DEFAULT_LINE_HEIGHT
96 arcade.draw_text("Kenney High Square Font",
97 start_x,
98 start_y,
99 arcade.color.BLACK,
100 DEFAULT_FONT_SIZE,
101 font_name="Kenney High Square")
102
103 start_y -= DEFAULT_LINE_HEIGHT
104 arcade.draw_text("Kenney Mini Square Font",
105 start_x, start_y,
106 arcade.color.BLACK,
107 DEFAULT_FONT_SIZE,
108 font_name="Kenney Mini Square")
109
110 start_y -= DEFAULT_LINE_HEIGHT
111 arcade.draw_text("Kenney Pixel Font",
112 start_x, start_y,
113 arcade.color.BLACK,
114 DEFAULT_FONT_SIZE,
115 font_name="Kenney Pixel")
116
117 start_y -= DEFAULT_LINE_HEIGHT
118 arcade.draw_text("Kenney Pixel Square Font",
119 start_x, start_y,
120 arcade.color.BLACK,
121 DEFAULT_FONT_SIZE,
122 font_name="Kenney Pixel Square")
123
124 start_y -= DEFAULT_LINE_HEIGHT
125 arcade.draw_text("Kenney Rocket Font",
126 start_x, start_y,
127 arcade.color.BLACK,
128 DEFAULT_FONT_SIZE,
129 font_name="Kenney Rocket")
130
131 start_y -= DEFAULT_LINE_HEIGHT
132 arcade.draw_text("Kenney Rocket Square Font",
133 start_x, start_y,
134 arcade.color.BLACK,
135 DEFAULT_FONT_SIZE,
136 font_name="Kenney Rocket Square")
137
138 start_y -= DEFAULT_LINE_HEIGHT
139 # When trying to use system fonts, it can be risky to specify
140 # only a single font because someone else's computer might not
141 # have it installed. This is especially true if they run a
142 # different operating system. For example, if you are on Windows
143 # and a friend has a mac or Linux, they might not have the same
144 # fonts. Your game could look different or broken on their computer.
145 # One way around that is to provide multiple options for draw_text
146 # to try. It will use the first one it finds, and use Arial as a
147 # default if it can't find any of them.
148 # In the example below, draw_text is given a tuple of names for very
149 # similar fonts, each of which is common on a different major
150 # operating systems.
151 arcade.draw_text("Times New Roman (Or closest match on system)",
152 start_x, start_y,
153 arcade.color.BLACK,
154 DEFAULT_FONT_SIZE,
155 font_name=(
156 "Times New Roman", # Comes with Windows
157 "Times", # MacOS may sometimes have this variant
158 "Liberation Serif" # Common on Linux systems
159 ))
160
161 start_y -= DEFAULT_LINE_HEIGHT
162 arcade.draw_text("Multi-Line\ntext using\n\\n characters.",
163 start_x, start_y,
164 arcade.color.BLACK,
165 DEFAULT_FONT_SIZE / 2,
166 multiline=True,
167 width=300)
168
169 start_y -= DEFAULT_LINE_HEIGHT * 1.5
170 arcade.draw_text("Wrapping really long text automatically to a new line. "
171 "The quick brown fox jumped over the lazy dogs.",
172 start_x,
173 start_y,
174 arcade.color.BLACK,
175 DEFAULT_FONT_SIZE / 2,
176 multiline=True,
177 width=300)
178
179 # --- Column 2 ---
180 start_x = 750
181 start_y = SCREEN_HEIGHT - DEFAULT_LINE_HEIGHT * 3
182 arcade.draw_text("Text Positioning:",
183 start_x,
184 start_y,
185 arcade.color.FRENCH_WINE,
186 DEFAULT_FONT_SIZE,
187 bold=True)
188
189 # start_x and start_y make the start point for the text.
190 # We draw a dot to make it easy too see the text in relation to
191 # its start x and y.
192 start_y -= DEFAULT_LINE_HEIGHT
193 arcade.draw_point(start_x, start_y, arcade.color.BARN_RED, 5)
194 arcade.draw_text("Default of 'baseline' and 'Left'",
195 start_x,
196 start_y,
197 arcade.color.BLACK,
198 DEFAULT_FONT_SIZE)
199
200 start_y -= DEFAULT_LINE_HEIGHT
201 arcade.draw_point(start_x, start_y, arcade.color.BARN_RED, 5)
202 arcade.draw_text("'bottom' and 'left'",
203 start_x,
204 start_y,
205 arcade.color.BLACK,
206 DEFAULT_FONT_SIZE,
207 anchor_x="left",
208 anchor_y="bottom")
209
210 start_y -= DEFAULT_LINE_HEIGHT
211 arcade.draw_point(start_x, start_y, arcade.color.BARN_RED, 5)
212 arcade.draw_text("'top' and 'left'",
213 start_x, start_y,
214 arcade.color.BLACK,
215 DEFAULT_FONT_SIZE,
216 anchor_x="left",
217 anchor_y="top")
218
219 start_y -= DEFAULT_LINE_HEIGHT * 2
220 arcade.draw_point(start_x, start_y, arcade.color.BARN_RED, 5)
221 arcade.draw_text("'baseline' and 'center'",
222 start_x, start_y,
223 arcade.color.BLACK,
224 DEFAULT_FONT_SIZE,
225 anchor_x="center",
226 anchor_y="baseline")
227
228 start_y -= DEFAULT_LINE_HEIGHT
229 arcade.draw_point(start_x, start_y, arcade.color.BARN_RED, 5)
230 arcade.draw_text("'baseline' and 'right'",
231 start_x,
232 start_y,
233 arcade.color.BLACK,
234 DEFAULT_FONT_SIZE,
235 anchor_x="right",
236 anchor_y="baseline")
237
238 start_y -= DEFAULT_LINE_HEIGHT
239 arcade.draw_point(start_x, start_y, arcade.color.BARN_RED, 5)
240 arcade.draw_text("'center' and 'center'",
241 start_x,
242 start_y,
243 arcade.color.BLACK,
244 DEFAULT_FONT_SIZE,
245 anchor_x="center",
246 anchor_y="center")
247
248 start_y -= DEFAULT_LINE_HEIGHT * 4
249 # start_x = 0
250 # start_y = 0
251 arcade.draw_point(start_x, start_y, arcade.color.BARN_RED, 5)
252 arcade.draw_text("Rotating Text",
253 start_x, start_y,
254 arcade.color.BLACK,
255 DEFAULT_FONT_SIZE,
256 anchor_x="center",
257 anchor_y="center",
258 rotation=self.text_angle)
259
260
261def main():
262 MyGame(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
263 arcade.run()
264
265
266if __name__ == "__main__":
267 main()